iOS SDK
Updated Mar 24, 2025
Overview of iOS SDK
Integrate server-side Google Tag Manager functionality into iOS applications with Stape sGTM iOS SDK. You can send data from your iOS apps to a server-side GTM container, facilitating advanced data processing and management. In the result, get more flexible tracking and analytics implementations!
This approach can enhance data privacy, improve performance, and provide more control over data collection and usage in iOS applications.
The Stape sGTM iOS SDK offers two key functionalities:
- it can create and dispatch events to a Data Client, with the SDK's API providing a simplified response model containing the Data Client's response.
- it integrates with Firebase, allowing for Firebase events to be converted into server-side Google Tag Manager events.
How to set up iOS SDK
Cocoapods
To install StapeSDK with Cocoapods just add this line to your Podfile: pod 'StapeSDK'
and run pod install
.
SPM
For now, SPM does not support packages with mixed ObjC/Swift code.
We'll add SPM support once this is included in SPM.
Feature is already in review, you can track progress here: https://forums.swift.org/t/se-0403-package-manager-mixed-language-target-support/66202
Connection
To use Stape SDK, you need to import it:
[object HTMLPreElement]
Then start it with config that provides credentials and connection settings. First create an instance of the Stape.Configuration
and pass it to the Stape.start()
method:
[object HTMLPreElement]
After that SDK is ready to use.
Sending events
To send an event you create instance of Stape.Event
:
[object HTMLPreElement]
and send it by passing to the Stape.send()
method:
[object HTMLPreElement]
Stape.send()
method provides a callback that is called after event got response and passed a result.
If event was successfully sent result contains Stape.EventResponse
instance.
Response provides a payload
property which is a dictionary with serialized key/value pairs got from the backend.
Note that response handler is called on a non-main thread, and you need to manually dispatch to the main thread if needed.
Stape.Event
struct provides a number of predefined keys for convenience. Keys are listed in Stape.Event.Keys
enum:
[object HTMLPreElement]
and can be used to compose event as follows:
[object HTMLPreElement]
Firebase hooking
To start listening to Firebase events, you need to call Stape.startFBTracking()
method after the call to Stape.start()
.
There is a handlers API to get responses for Firebase events dispatched to Stape Data Client.
It consists of a pair of methods to add and remove handler closures identified by keys:
[object HTMLPreElement]
Logging
Stape SDK uses Apple os.log facility to log its actions.
You can use com.stape.logger
subsystem to filter Stape SDK logs.
To learn more about Apple structured logging, please refer to the official documentation: https://developer.apple.com/documentation/os/logging`
Stape Data Client in server GTM
1. Download Data Client from GitHub.
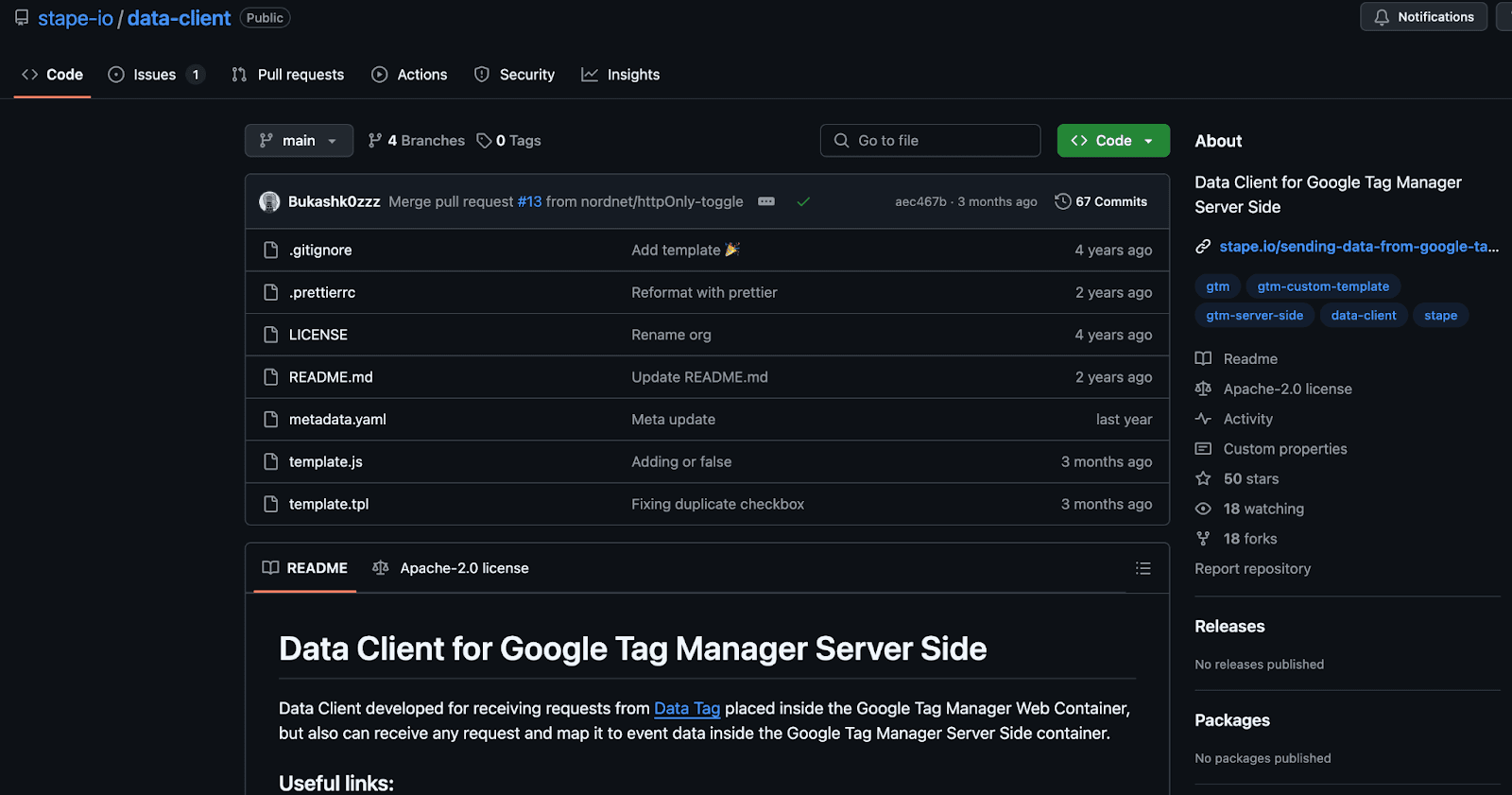
2. Go to your server GTM container ➝ Templates ➝ Add new Client template and select .tpl file that you loaded from GitHub.
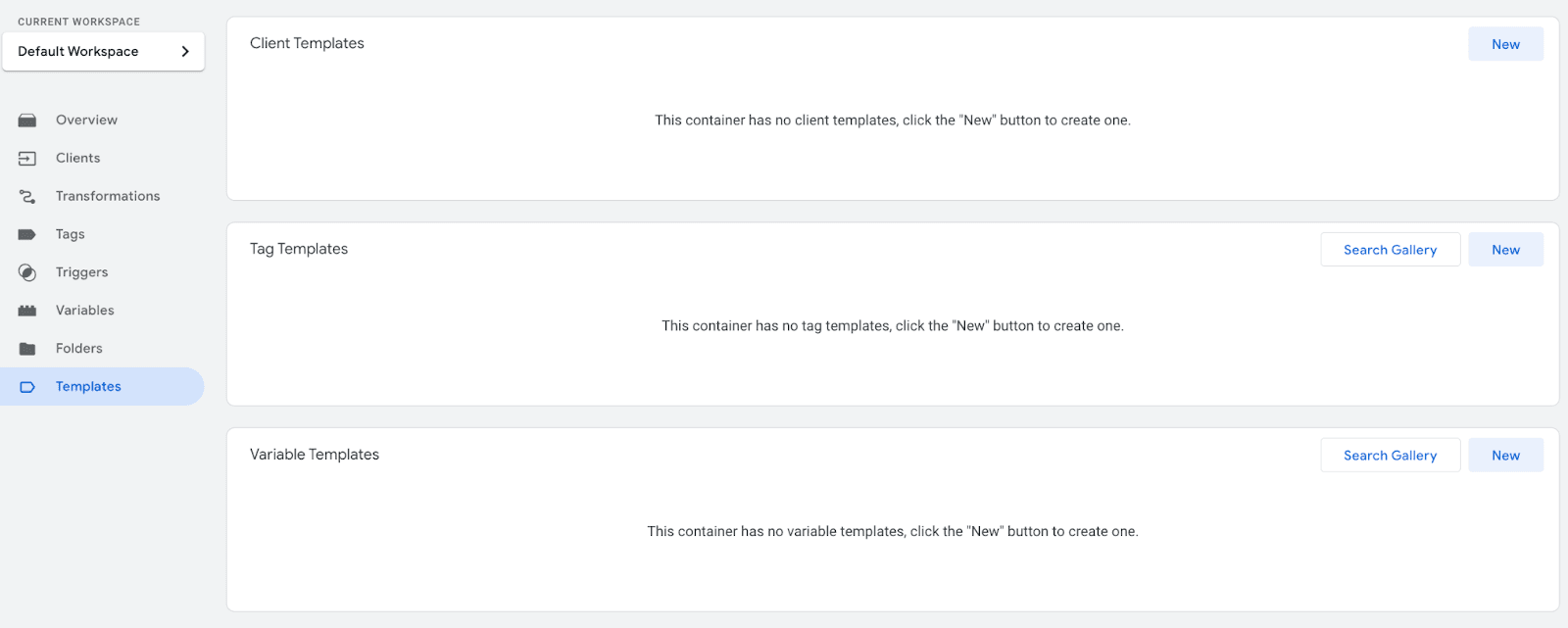
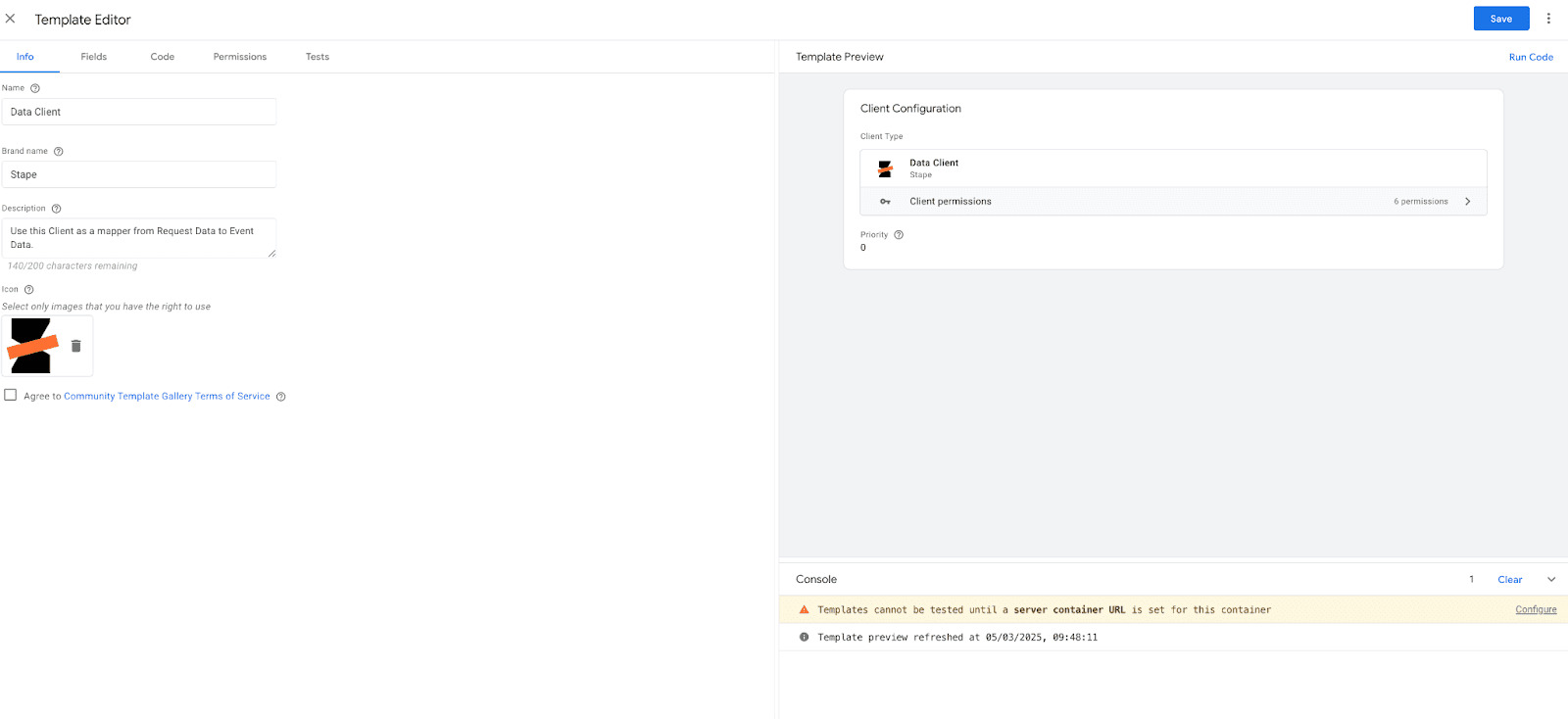
3. Go to ‘Clients’ section on your server GTM container ➝ New client ➝ Add Data Client from templates.
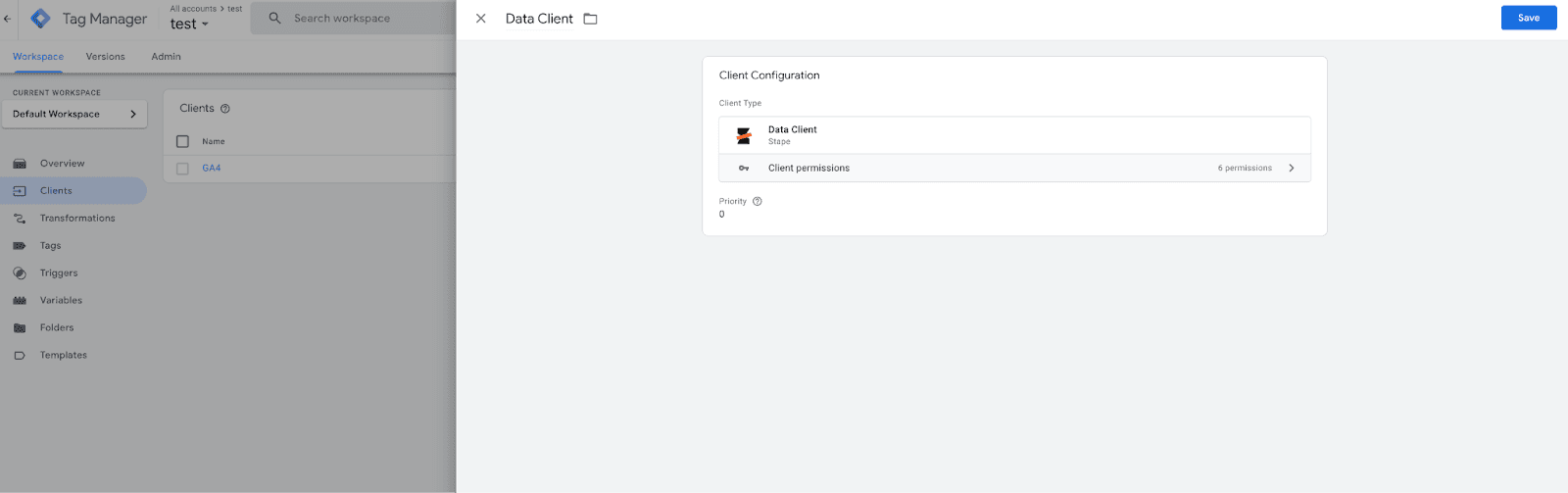
4. Submit changes on your server GTM container to make it live.
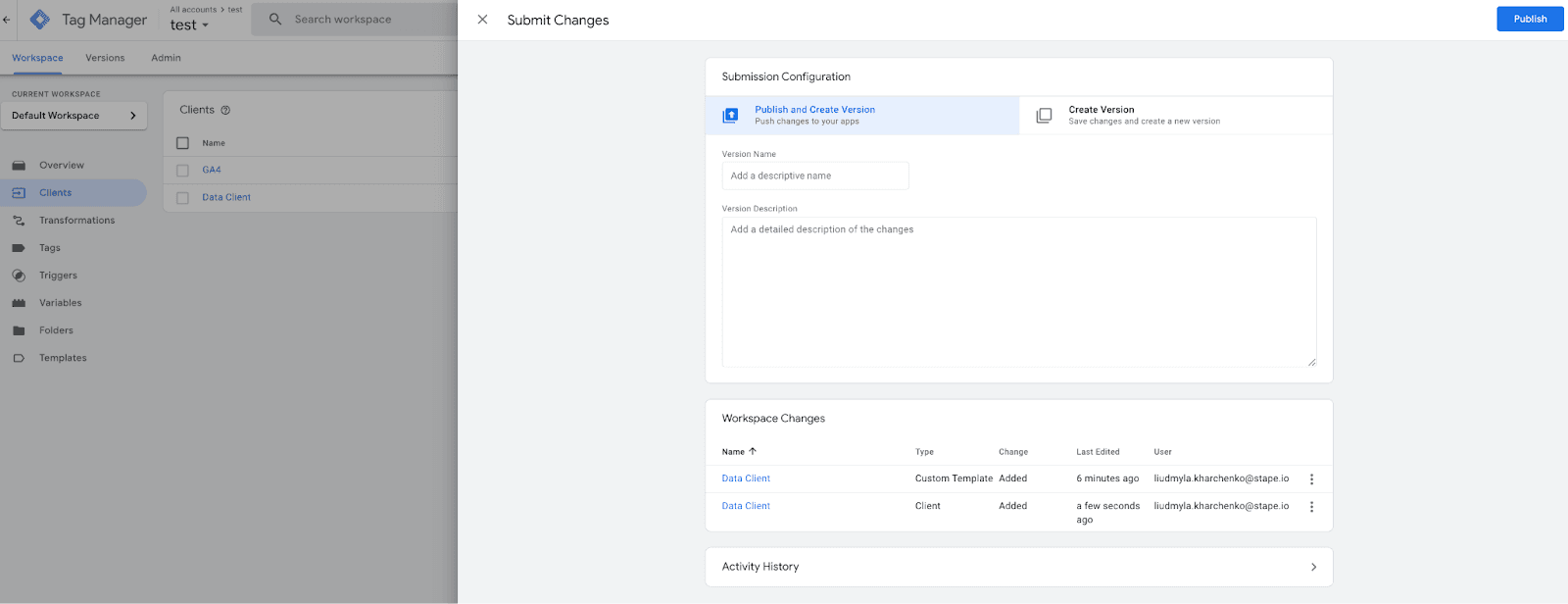
How to add iOS SDK to your project
1. Ensure you've installed the Stape SDK via CocoaPods:
pod 'StapeSDK'
2. Run it through.
pod install
3. Initialize SDK in your project.
import StapeSDK
@main
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
// Initialize Stape SDK with your domain
if let url = URL(string: "https://gtm.example.com") {
let configuration = Stape.Configuration(domain: url)
Stape.start(configuration: configuration)
}
return true
}
}
4. Replace "gtm.example.com" with your actual sGTM container domain.
5. Create and send event.
import StapeSDK
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Send "add_to_cart" event
sendAddToCartEvent()
}
func sendAddToCartEvent() {
// Define event payload
let payload: [String: Any] = [
"value": 68,
"currency": "USD",
"page_hostname": "stape.io",
"page_location": "http://stape.io",
"user_data": [
"email": sha256("jhonn@doe.com") // Hash email for privacy
]
]
// Create event
let event = Stape.Event(name: "add_to_cart", payload: payload)
// Send event
Stape.send(event: event) { result in
switch result {
case .success(let response):
print("✅ Event Sent: \(response.payload)")
case .failure(let error):
print("❌ Error: \(error.localizedDescription)")
}
}
}
// SHA256 hashing function
func sha256(_ input: String) -> String {
guard let data = input.data(using: .utf8) else { return "" }
var hash = [UInt8](repeating: 0, count: Int(CC_SHA256_DIGEST_LENGTH))
data.withUnsafeBytes {
_ = CC_SHA256($0.baseAddress, CC_LONG(data.count), &hash)
}
return hash.map { String(format: "%02x", $0) }.joined()
}
}
Note! Ensure you have the CommonCrypto library imported for the SHA256 hashing function. |
Firebase hooking
To start listening to Firebase events, you need to call Stape.startFBTracking() method after the call to Stape.start().
There is a handlers API to get responses for Firebase events dispatched to Stape Data Client.
It consists of a pair of methods to add and remove handler closures identified by keys:
public static func addFBEventHandler(_ handler: @escaping Completion, forKey key: String)
public static func removeFBEventHandler(forKey key: String)
How to test the setup
The server GTM preview only displays incoming requests sent from the tab adjacent to the Chrome browser where the preview mode runs. So, if you run sGTM preview and send a request through SDK, you will not see it in preview mode, although this does not prevent it from working.
You need to use the power-up sGTM Preview Header config to view and debug SDK requests. We have a blog post describing setting up and debugging incoming webhooks in the sGTM.
If you are configuring this on a live container that receives a lot of hits, activating the HTTP header may not be a good idea. It will display all requests coming to the server in the preview, which can lead to performance issues for the container if the preview receives hundreds or thousands of requests per second.
In such a case, it would be a good idea to start a ‘staging’ container on a free plan, where everything is configured and debugged, and then transfer that to a live container and the live URL of your server GTM.
The most common problem when using the SDK is getting a 400 error
400 error means that no client has processed your request. In this case, make sure that Data Client is installed on your server GTM container, and that these changes are omitted (check in the Versions tab that the latest version of the container is live).
Also make sure that the /path to which you send requests is specified in the Data Client (if you use something other than the standard /data).