Android SDK
Updated Mar 24, 2025
Overview of Android SDK
Stape sGTM Android SDK enables the integration of server-side Google Tag Manager into the Android apps. It can help with efficient tracking and data collection capabilities, improving the analytics and marketing strategies within the application.
Android SDK from Stape has these features:
- it enables the creation and dispatch of events to a Data Client. The SDK's API then returns a response from the Data Client, which is organized into a simple model with serialized response fields.
- it integrates with Firebase to facilitate the conversion of Firebase events into server-side Google Tag Manager events.
How to set up Android SDK
Add the dependency to your build.gradle file:
[object HTMLPreElement]
Make use you using the latest version of Stape SDK.
Initialization
To use the Stape SDK, you need to create an instance of the Stape
class.
The instance can be created using the Stape.withOption
method.
The method takes an Options
object as a parameter.
[object HTMLPreElement]
The Options
object contains data for correct SDK initialization and requires only one parameter - domain
.
The domain
parameter is a domain name of your sGTM container instance.
Do not include https://
or http://
schemas.
Please do not override the default Options
values if you are not sure what you are doing.
Sending events
After the Stape instance is created, you can send events to the Stape Data Client.
Stape SDK allows sending data in a couple of ways:
- Using a Map collection;
- Using an EventData class.
These two ways are equivalent, and you can use any of them:
[object HTMLPreElement]
Please extend the EventData
class if you need to send any other data.
Tracking Firebase events
Stape SDK allows to decorate Firebase Analytics instance and deliver all sent events to the Stape Data Client as well.
For this purpose you need to use FirebaseAnalyticsAdapter
class.
[object HTMLPreElement]
The FirebaseAnalyticsAdapter
provides absolutely the same API as the Firebase Analytics instance.
It means that you can replace the provided types without a lot of changes.
Trying sample app
If you desire to try the sample app, please follow the next steps:
- Clone the repository;
- Open the project in Android Studio;
- Create a Firebase project with enabled Analytics;
- Generate your own
- Create
- Rebuild the app.
Stape Data Client in server GTM
1. Download Data Client from GitHub.
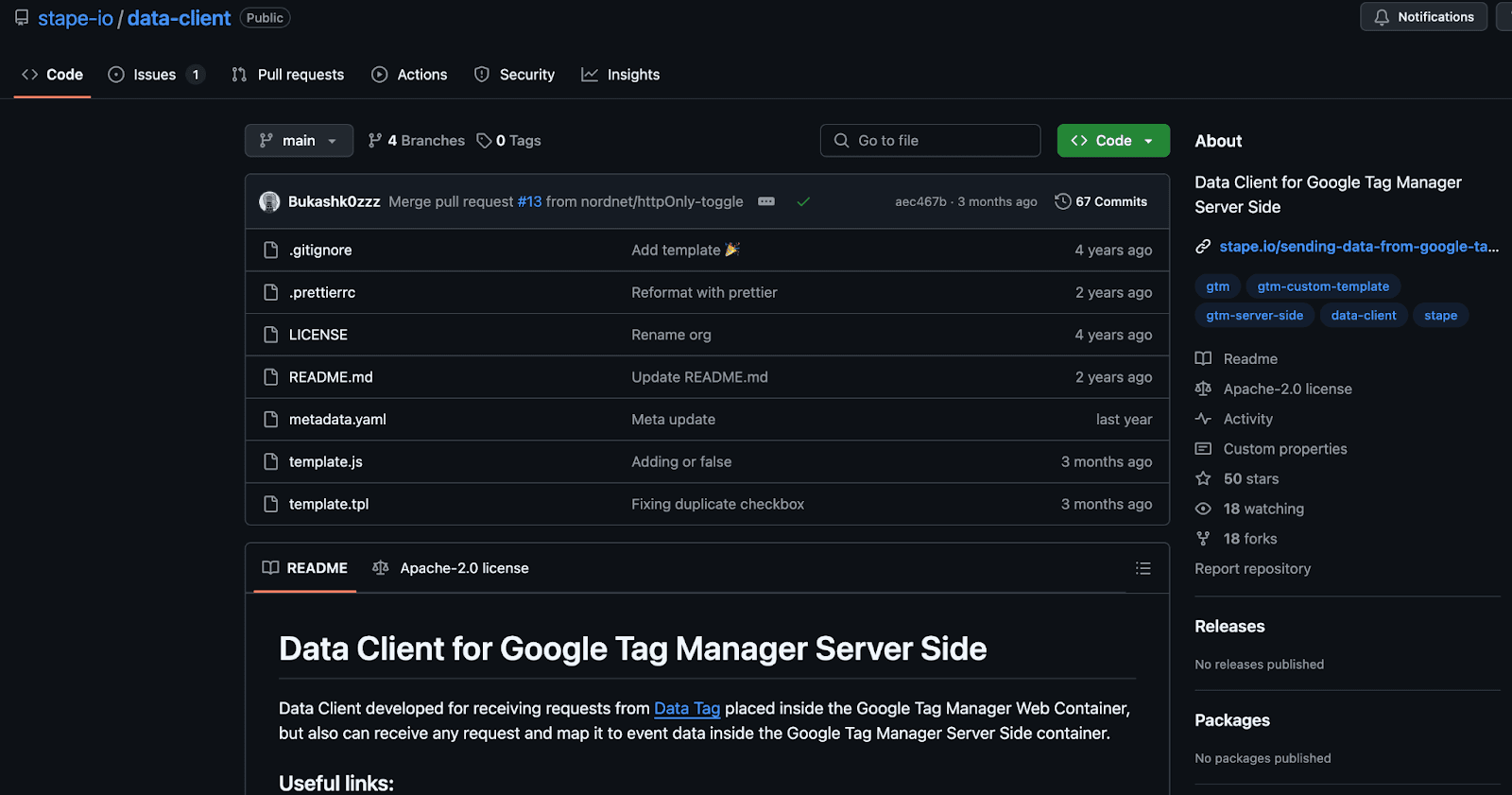
2. Go to your server GTM container ➝ Templates ➝ Add new Client template and select .tpl file that you loaded from GitHub.
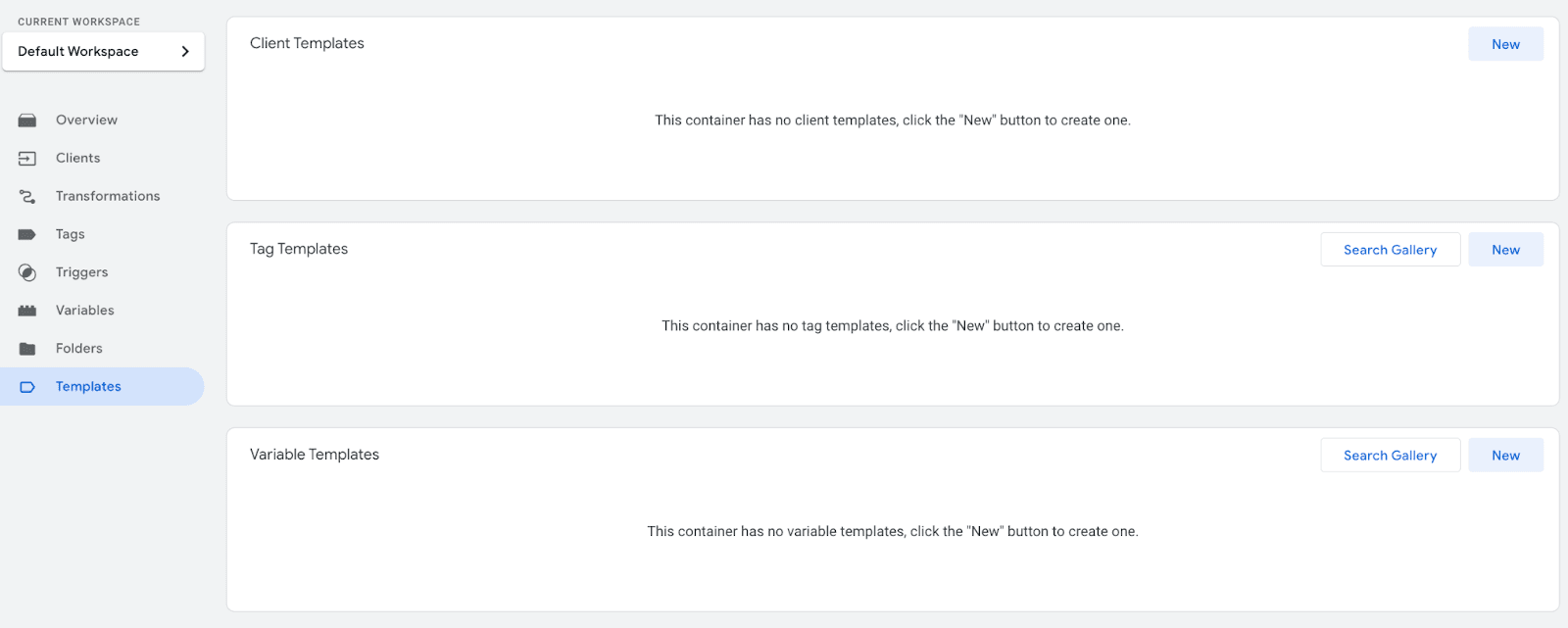
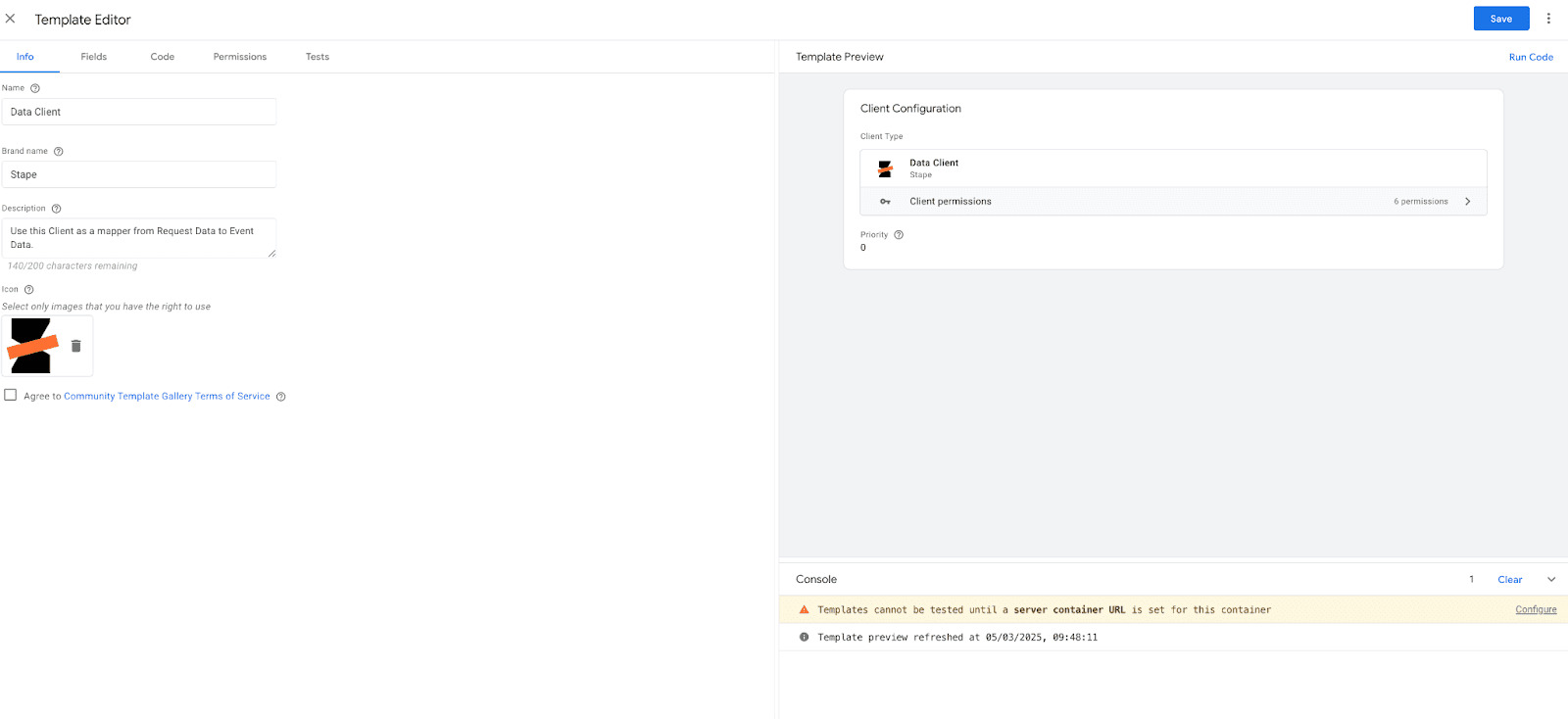
3. Go to ‘Clients’ section on your server GTM container ➝ New client ➝ Add Data Client from templates.
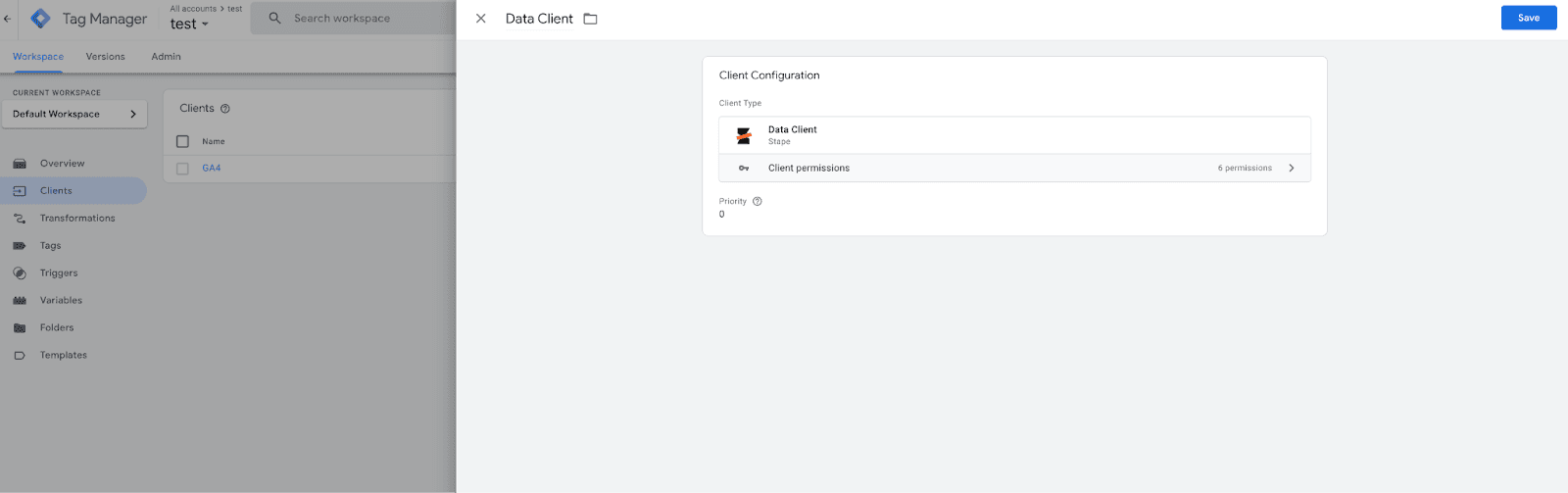
4. Submit changes on your server GTM container to make it live.
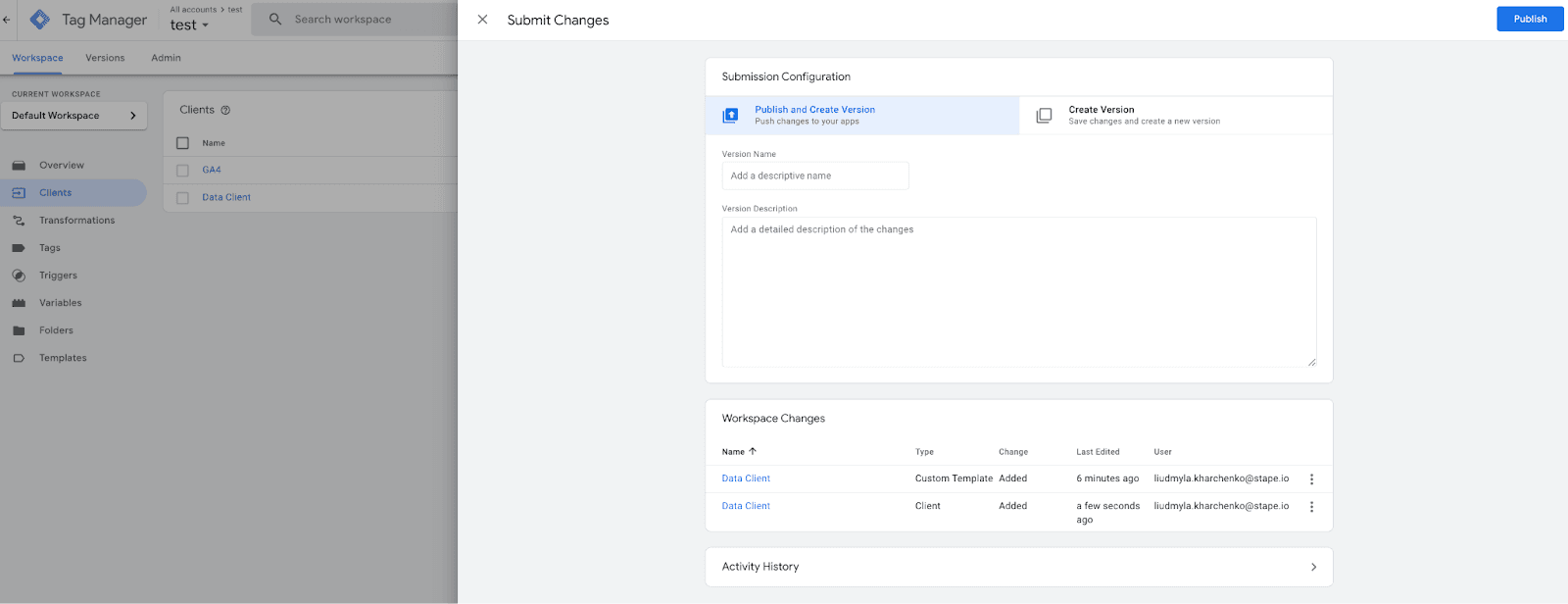
How to add Android SDK to your project
1. Add the Stape SDK dependency to your build.gradle file.
implementation 'io.stape:android-sgtm:1.0'
2. Initialize the SDK in your Application class.
import android.app.Application;
import io.stape.sgtm.Options;
import io.stape.sgtm.Stape;
public class MyApp extends Application {
@Override
public void onCreate() {
super.onCreate();
Options options = new Options("gtm.example.com");
Stape stape = Stape.withOptions(options);
}
}
3. Replace "gtm.example.com" with your actual sGTM container domain, excluding https:// or http:// schemas.
4. Create and send the event from your activity or fragment.
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import io.stape.sgtm.Stape;
import java.util.HashMap;
import java.util.Map;
public class MainActivity extends AppCompatActivity {
private Stape stape;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Initialize Stape instance
stape = Stape.getInstance();
// Send "Add to Cart" event
sendAddToCartEvent();
}
private void sendAddToCartEvent() {
// Create event data
Map eventData = new HashMap<>();
eventData.put("value", 68);
eventData.put("currency", "USD");
eventData.put("page_hostname", "stape.io");
eventData.put("page_location", "http://stape.io");
eventData.put("user_data", getUserData());
// Send event
stape.sendEvent("add_to_cart", eventData);
}
private Map getUserData() {
Map userData = new HashMap<>();
userData.put("email", sha256("jhonn@doe.com"));
return userData;
}
private String sha256(String input) {
try {
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] hash = digest.digest(input.getBytes(StandardCharsets.UTF_8));
StringBuilder hexString = new StringBuilder();
for (byte b : hash) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) hexString.append('0');
hexString.append(hex);
}
return hexString.toString();
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException(e);
}
}
}
How to test the setup
The server GTM preview only displays incoming requests sent from the tab adjacent to the Chrome browser where the preview mode runs. So, if you run sGTM preview and send a request through SDK, you will not see it in preview mode, although this does not prevent it from working.
You need to use the power-up sGTM Preview Header config to view and debug SDK requests. We have a blog post describing setting up and debugging incoming webhooks in the sGTM.
If you are configuring this on a live container that receives a lot of hits, activating the HTTP header may not be a good idea. It will display all requests coming to the server in the preview, which can lead to performance issues for the container if the preview receives hundreds or thousands of requests per second.
In such a case, it would be a good idea to start a ‘staging’ container on a free plan, where everything is configured and debugged, and then transfer that to a live container and the live URL of your server GTM.
The most common problem when using the SDK is getting a 400 error
400 error means that no client has processed your request. In this case, make sure that Data Client is installed on your server GTM container, and that these changes are omitted (check in the Versions tab that the latest version of the container is live).
Also make sure that the /path to which you send requests is specified in the Data Client (if you use something other than the standard /data).